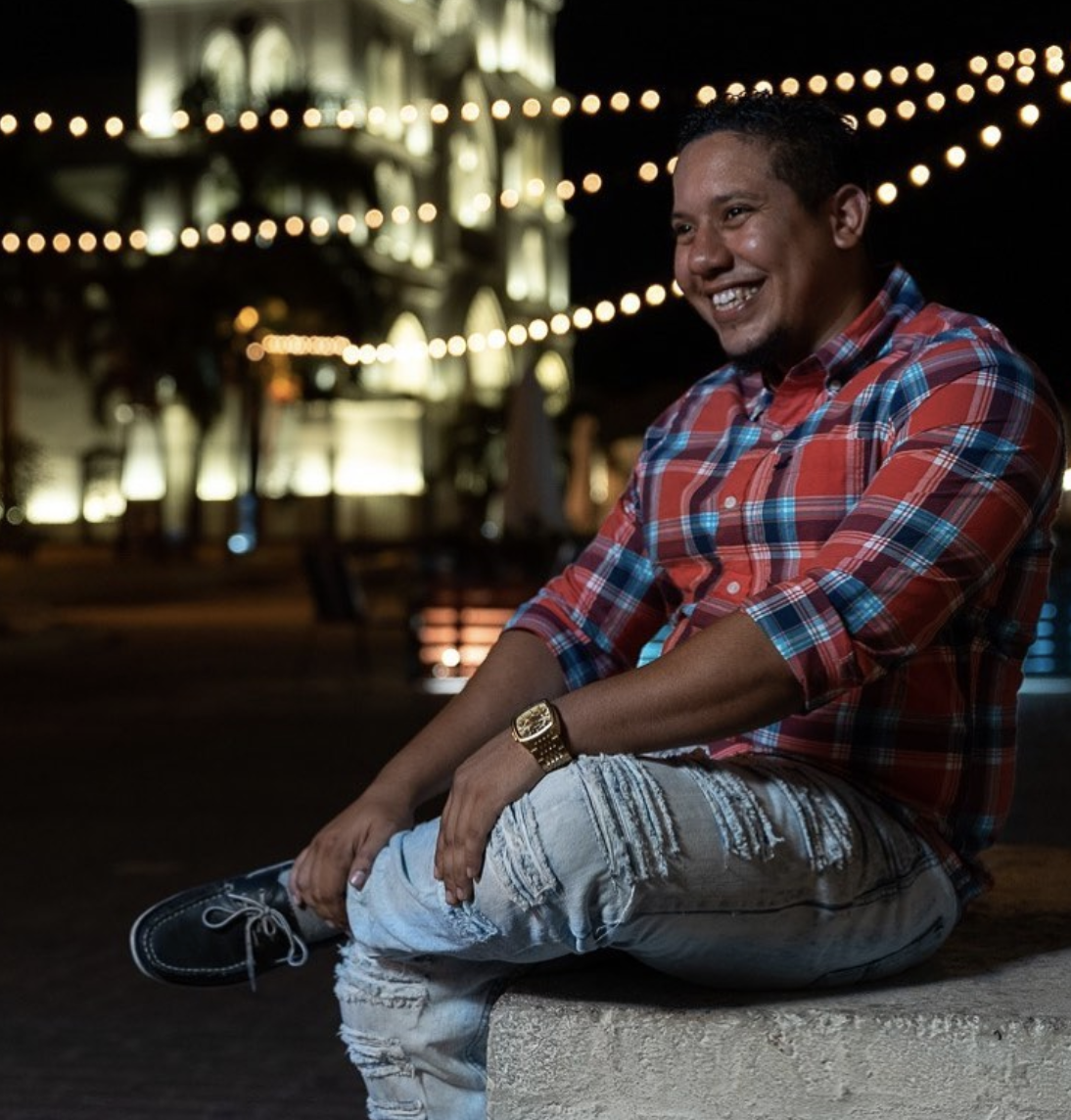
Fermin Perdomo
Full Stack Developer
© 2025 Fermin's Portfolio | All rights reserved.

From Salesforce to Laravel: A Guide to Successful Lead Management Integration
This blog post will examine how to integrate lead management from Salesforce to Laravel successfully.1. Create a Salesforce Developer AccountThe first step in integrating Salesforce's lead management into your Laravel application is to create a Salesforce Developer account. This account will give you access to the Salesforce API, essential for integrating with Laravel.To create a Salesforce Developer account, visit the website and sign up for a free account. Once you've made your account, you must create a new connected app in Salesforce. This app will give your Laravel application access to the Salesforce API.2. Install the Salesforce SDK for LaravelYou must install the Salesforce SDK for PHP to integrate Salesforce's lead management into your Laravel application. This SDK provides tools and libraries that allow you to interact with the Salesforce API from your Laravel application.To install the Salesforce SDK for PHP, you can use Composer, a dependency manager for PHP. In your Laravel application's root directory, run the following command:This command will install the Salesforce SDK for PHP in your Laravel application.The package will automatically register the service provider and
Forrest
alias for Laravel >=5.5
. For earlier versions, add the service provider and alias to your config/app.php
file:3. ConfigurationYou will need a configuration file to add your credentials. Publish a config file using the artisan
command:This will publish a config/forrest.php
file that can switch between authentication types and other settings.After adding the config file, update your .env
to include the following values (details for getting a consumer key and secret are outlined below):4. SetupCreating authentication routesIn this case, we will use the Web Server authentication flow5. Basic usageAfter authentication, your app will store an encrypted authentication token which can be used to make API requests.Query a record:Sample result:If you are querying more than 2000 records, your response will include the following:Call Forrest::next($nextRecordsUrl) to return the next 2000 records.Here's an example of how to retrieve all leads from Salesforce:6. Create Leads in SalesforceIn addition to retrieving leads from Salesforce, you can also create new leads in Salesforce using the API. Here's an example of how to create a new lead in Salesforce7. Update a Salesforce leadUpdate a record with the PUT method; if the external Id doesn't exist, it will insert a new one.ConclusionIn this blog post, we've explored how to integrate lead management from Salesforce to Laravel successfully. Following these steps, you can leverage Salesforce's powerful lead management capabilities in your Laravel application. With access to the Salesforce API, you can retrieve, create, and update leads in Salesforce, providing a seamless experience for your team and customers.