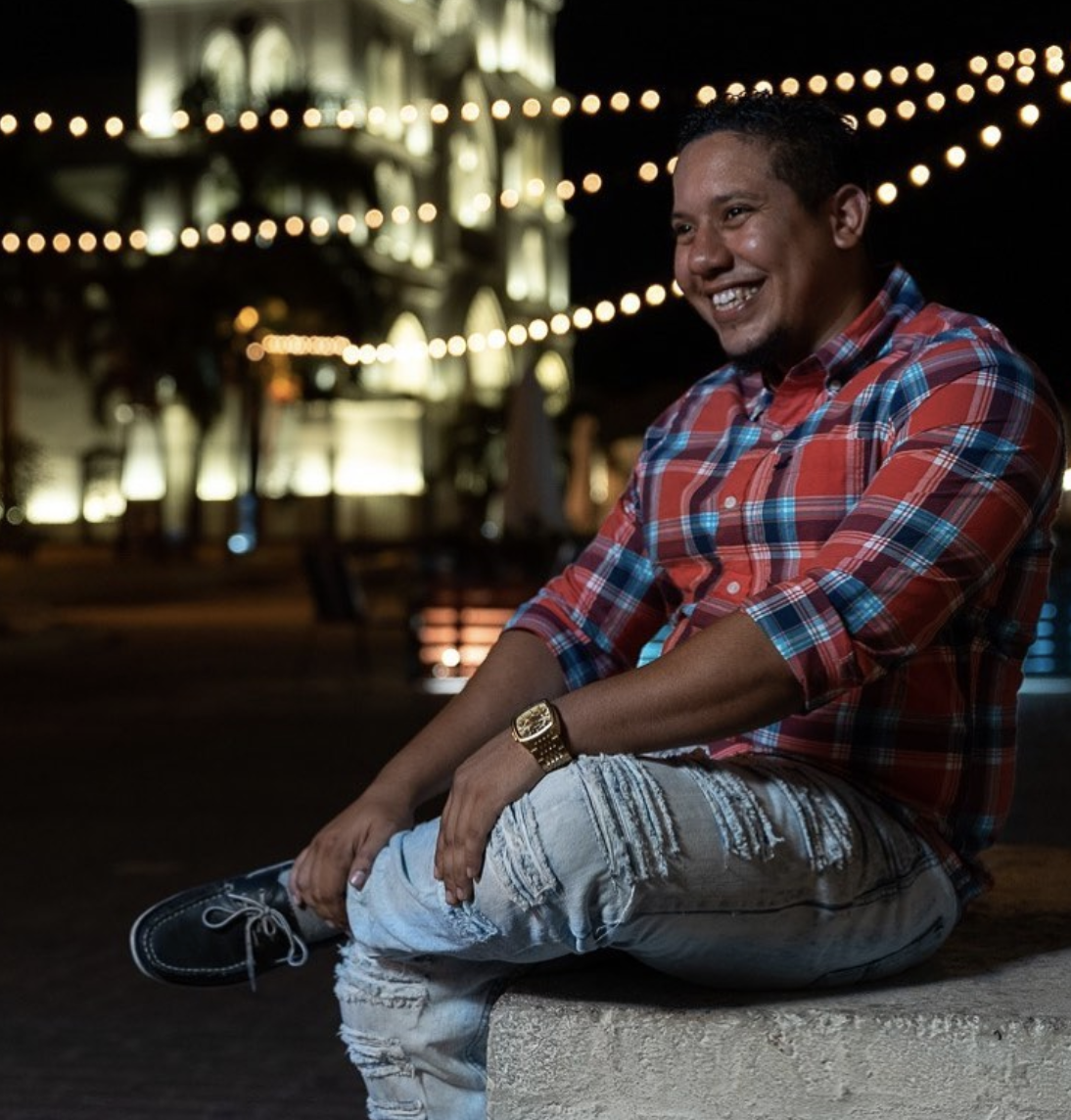
Fermin Perdomo
Full Stack Developer
© 2025 Fermin's Portfolio | All rights reserved.

How to implement the bubble sort in PHP
How does bubble sort work?The basic idea of bubble sort is to iterate through the array, comparing adjacent elements and swapping them if necessary. This process is repeated until the entire array is sorted.Here is an example of how bubble sort works on an array of integers:
The first pass of the bubble sort algorithm compares the first two elements, 5
and 3
. Since 3
is smaller than 5
, they are swapped:
5
and 8
. Since they are already in the correct order, they are not swapped.The third pass compares the next two elements, 8
and 2
. Since 2
is smaller than 8
, they are swapped:The fourth pass compares the next two elements, 8
and 1
. Since 1
is smaller than 8
, they are swapped:The fifth pass compares the final two elements, 8
and 4
. Since 4
is smaller than 8
, they are swapped:At this point, the array is not fully sorted, so the bubble sort algorithm repeats the process, starting at the beginning of the array again.The sixth pass compares the first two elements, 3
and 5
. Since they are already in the correct order, they are not swapped.The seventh pass compares the next two elements, 5
and 2
. Since 2
is smaller than 5
, they are swapped:The eighth pass compares the next two elements, 5
and 1
. Since 1
is smaller than 5
, they are swapped:The ninth pass compares the next two elements, 5
and 4
. Since 4
is smaller than 5
, they are swapped:The tenth pass compares the final two elements, 5
and 8
. Since 8
is larger than 5
, they are not swapped. At this point, the array is fully sorted, and the bubble sort algorithm ends. The final sorted array is:How to implement bubble sort inThis function takes an array as input and returns a new array with the elements sorted in ascending order. The basic idea of bubble sort is to repeatedly iterate through the array, comparing adjacent elements and swapping them if they are in the wrong order. The inner loop starts at the beginning of the array and compares each pair of adjacent elements, swapping them if necessary. The outer loop then repeats this process for the next element, until the entire array is sorted.Here is an example of how you can use this function:ConclusionBubble sort has a time complexity of O(n^2) in the worst case, so it is not the most efficient sorting algorithm for large arrays. However, it is simple to understand and implement, and can be useful for small arrays or as a learning exercise.