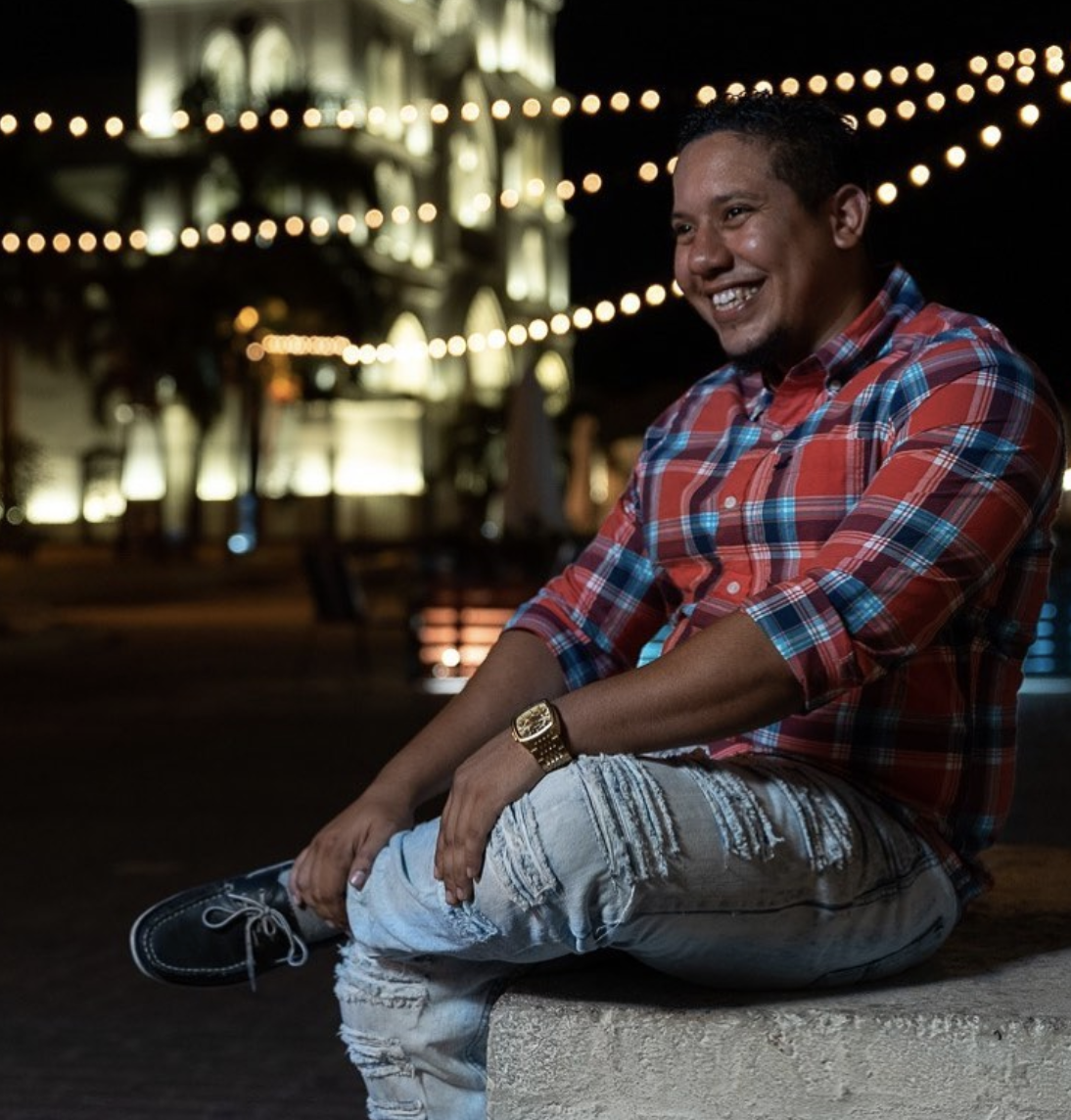
Fermin Perdomo
Full Stack Developer
© 2025 Fermin's Portfolio | All rights reserved.
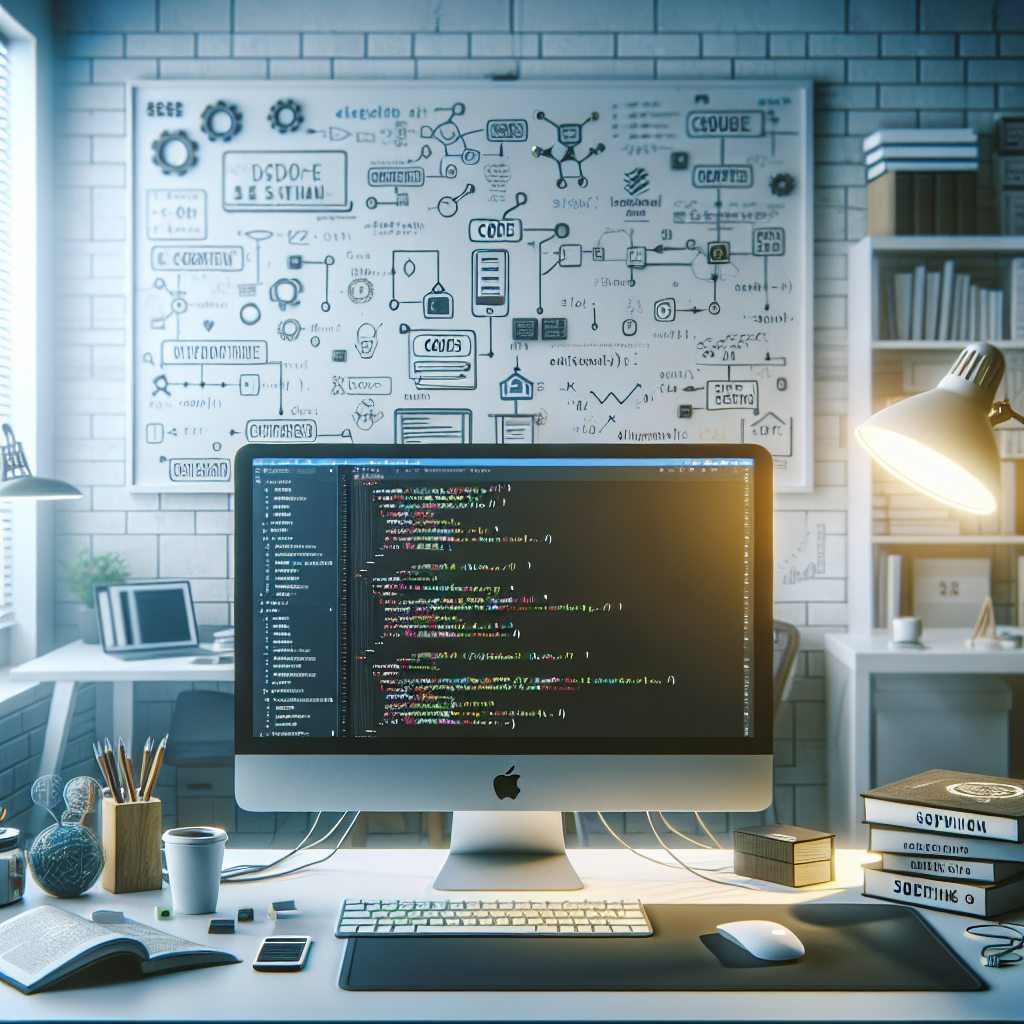
Simplify Your JSON File Processing with Simple JSON in PHP
One of the key features of Simple JSON is its use of generators to minimize memory usage, even when dealing with large files. This means you can process your JSON files quickly and efficiently, without worrying about running out of memory.Here's an example of how to read a JSON file with Simple JSON:InstallationYou can install the package using composer:composer require diamond-dove/simple-jsonUsageReading a JSON fileTo read a JSON file, you can use the following code:Writing a JSON fileTo write a JSON file, you can use the following code:The file at
$pathToJson
will contain: