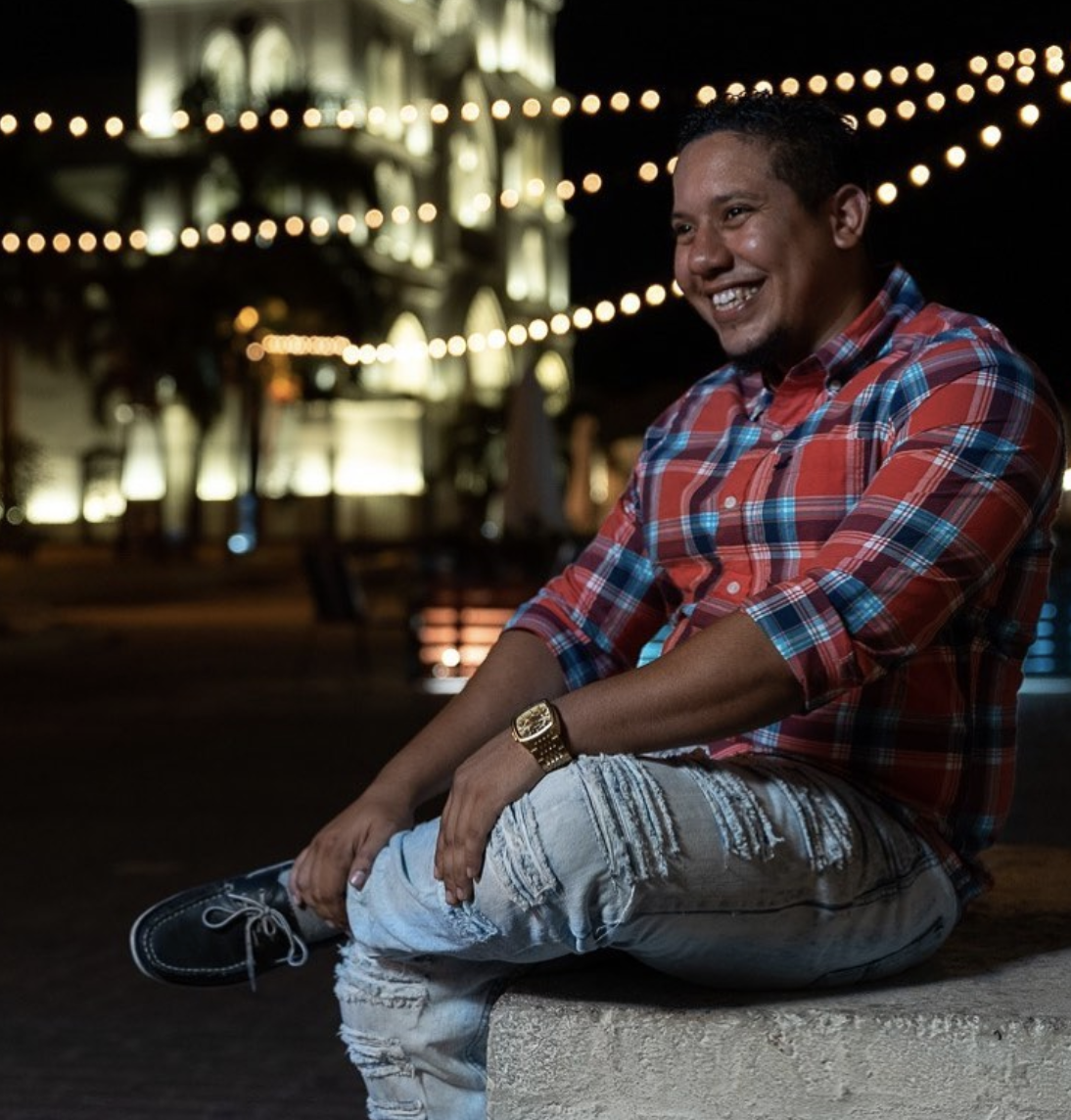
Fermin Perdomo
Full Stack Developer
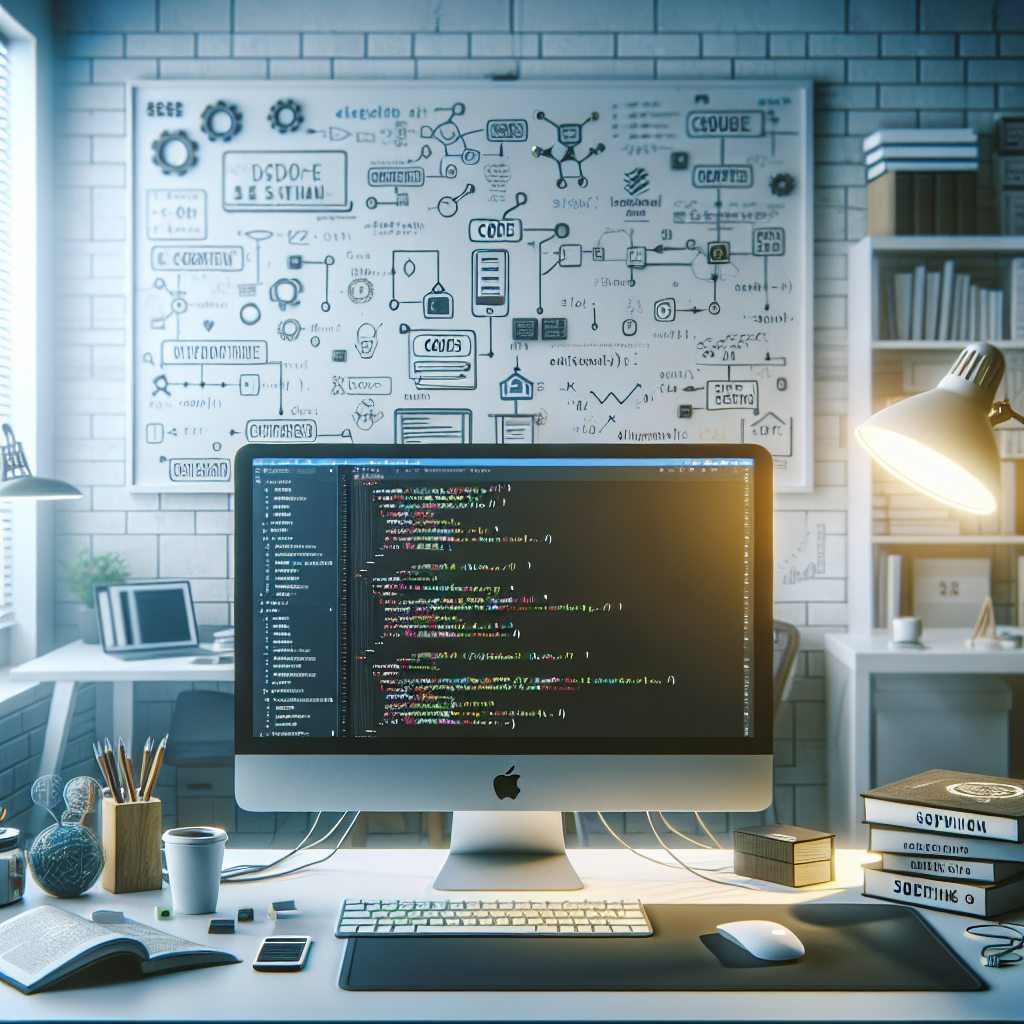
Learn How to Use PHP Generators for Data Iteration
A generator is a special type of function in PHP that allows you to create an iterable set of data without having to store the entire set in memory at once. This can be useful in situations where you need to work with large datasets or when you want to generate data on the fly.
Here's an example of a simple generator function in PHP:
function generateNumbers($start, $end) {
for ($i = $start; $i <= $end; $i++) {
yield $i;
}
}
To use this generator, you can use a foreach loop like this:
foreach (generateNumbers(1, 10) as $number) {
echo $number . "\n";
}
This would output the numbers from 1 to 10, one per line.
Generators have several advantages over regular functions. For one, they are more memory efficient because they only generate and return one value at a time, rather than creating an entire array of values up front. This can be particularly useful when working with large datasets that would otherwise consume a lot of memory.
Generators are also easier to write and read than other iterable data structures like arrays or objects, which can make them a more convenient choice in certain situations.