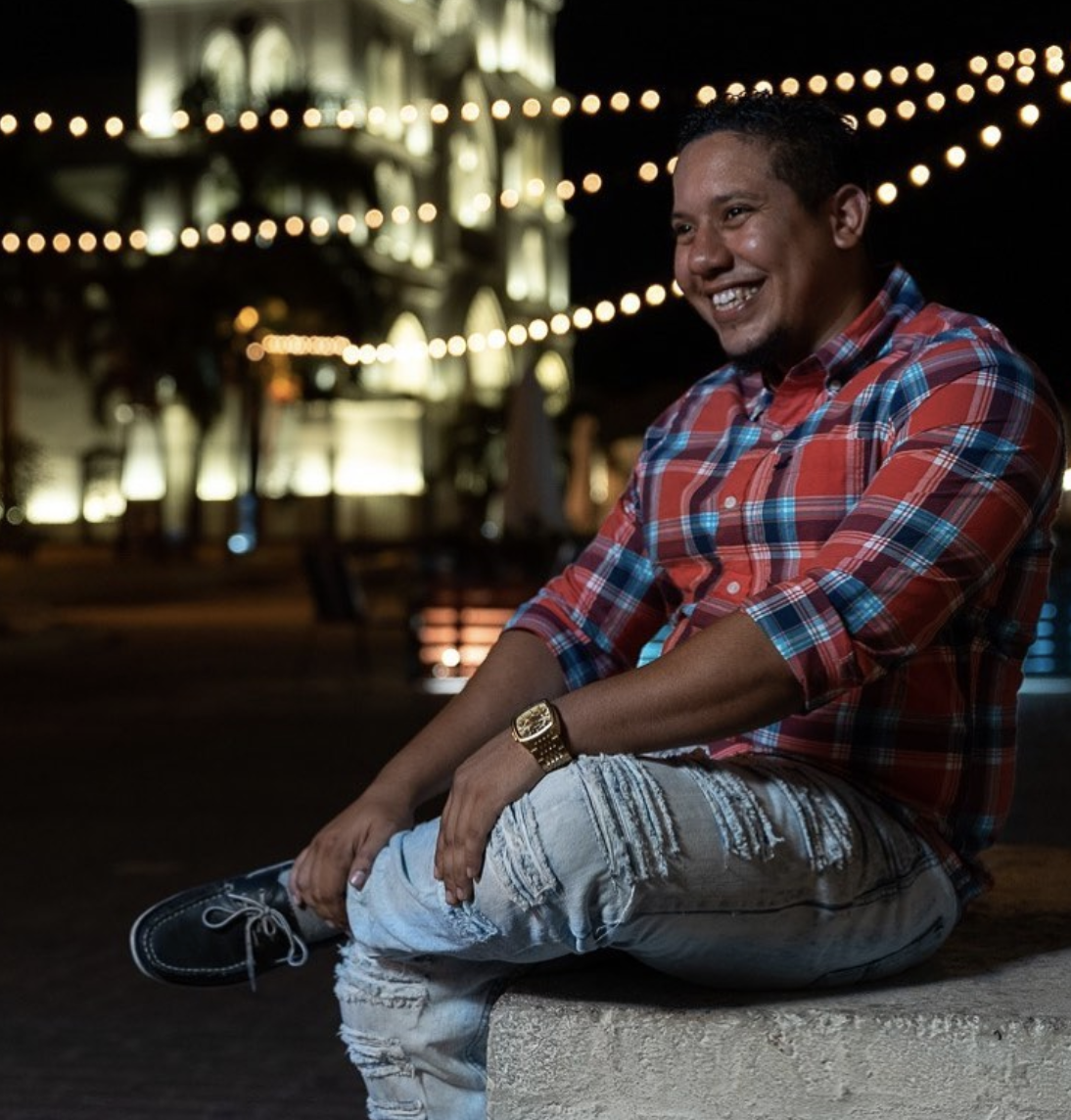
Fermin Perdomo
Full Stack Developer
© 2025 Fermin's Portfolio | All rights reserved.
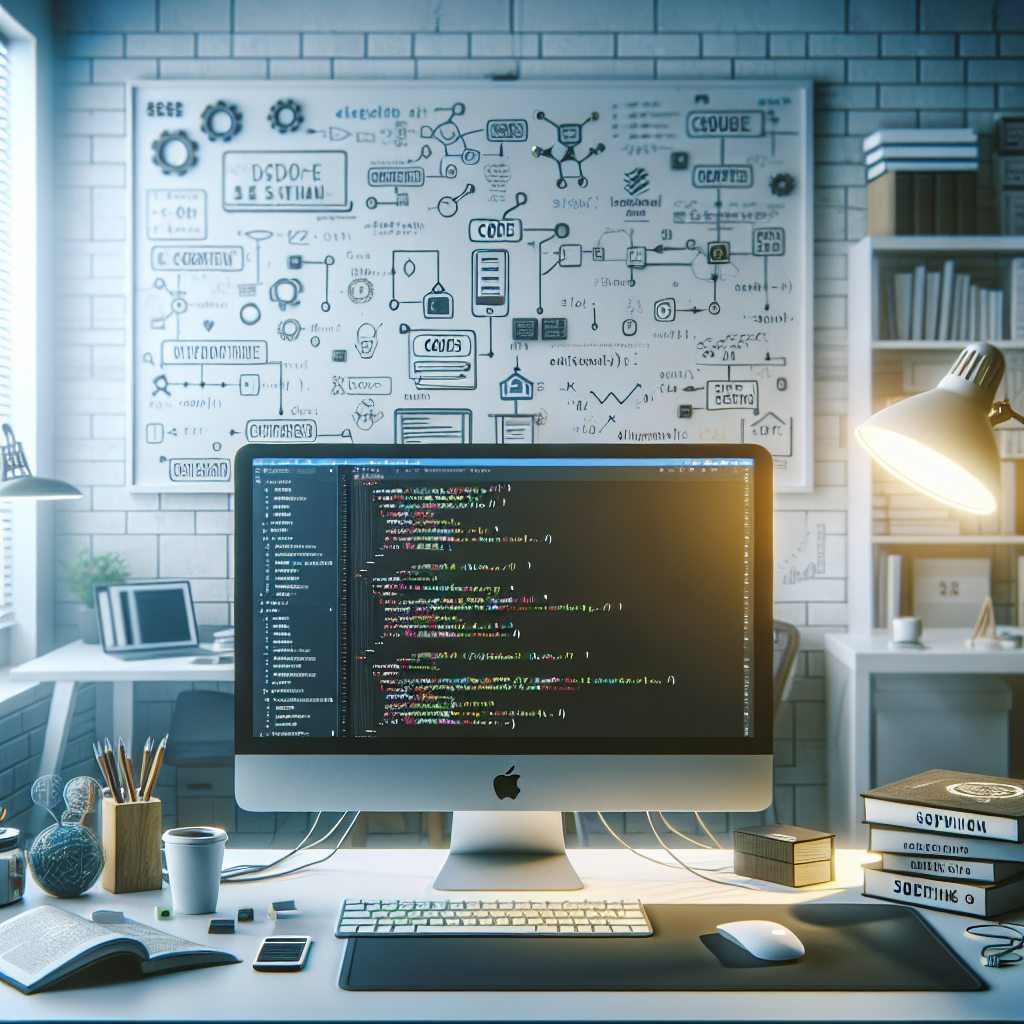
How I create a invoice to PDF
In this post, I will teach you how to generate a pdf from your invoice data.
The loadView
method is commonly used with PDF generation packages like barryvdh/laravel-dompdf to convert a Blade view into a PDF document.
Here’s a step-by-step example of how to use loadView
:
1. Install the Required Package
If you haven't already, install barryvdh/laravel-dompdf:
composer require barryvdh/laravel-dompdf
2. Set Up the Controller to Use loadView
In your controller, you can use loadView
to load a Blade view and generate a PDF.
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Barryvdh\DomPDF\Facade\Pdf;
use App\Models\Order;
class InvoiceController extends Controller
{
public function downloadInvoice($orderId)
{
// Fetch order data from the database
$order = Order::with('user', 'items')->findOrFail($orderId);
// Load the Blade view into PDF
$pdf = Pdf::loadView('invoices.invoice', compact('order'));
// Download the generated PDF
return $pdf->download('invoice_' . $order->id . '.pdf');
}
}
3. Create the Blade View for the Invoice
<!DOCTYPE html>
<html>
<head>
<title>Invoice #{{ $order->id }}</title>
<style>
body { font-family: Arial, sans-serif; }
.invoice-box { max-width: 800px; margin: auto; padding: 30px; border: 1px solid #eee; }
table { width: 100%; line-height: inherit; text-align: left; border-collapse: collapse; }
table td { padding: 5px; vertical-align: top; }
table tr.heading td { background: #eee; border-bottom: 1px solid #ddd; font-weight: bold; }
table tr.item td { border-bottom: 1px solid #eee; }
table tr.total td { border-top: 2px solid #eee; font-weight: bold; }
</style>
</head>
<body>
<div class="invoice-box">
<h2>Invoice #{{ $order->id }}</h2>
<p>Date: {{ $order->created_at->format('d/m/Y') }}</p>
<p>Customer: {{ $order->user->name }}</p>
<table>
<tr class="heading">
<td>Item</td>
<td>Quantity</td>
<td>Price</td>
</tr>
@foreach($order->items as $item)
<tr class="item">
<td>{{ $item->name }}</td>
<td>{{ $item->pivot->quantity }}</td>
<td>${{ number_format($item->pivot->price, 2) }}</td>
</tr>
@endforeach
<tr class="total">
<td></td>
<td>Total:</td>
<td>${{ number_format($order->total, 2) }}</td>
</tr>
</table>
</div>
</body>
</html>
4. Define the Route
Add the route to your web.php
file:
<?php
use App\Http\Controllers\InvoiceController;
Route::get('/invoice/{orderId}/download', [InvoiceController::class, 'downloadInvoice']);
5. Access the PDF
Now, visit:
http://your-app-url/invoice/1/download
This will generate and download a PDF file named invoice_1.pdf
based on the Blade template.