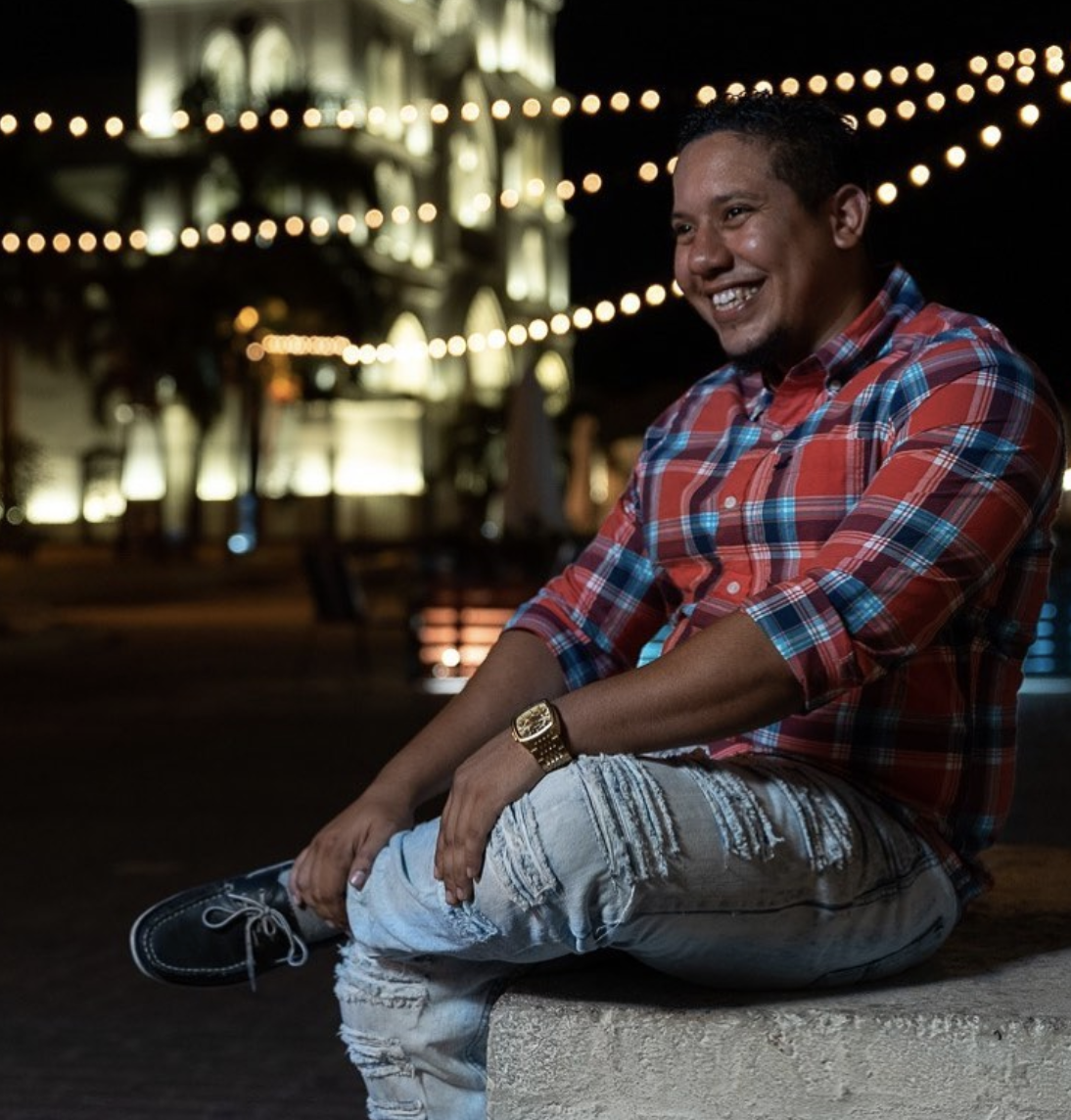
Fermin Perdomo
Full Stack Developer
© 2025 Fermin's Portfolio | All rights reserved.
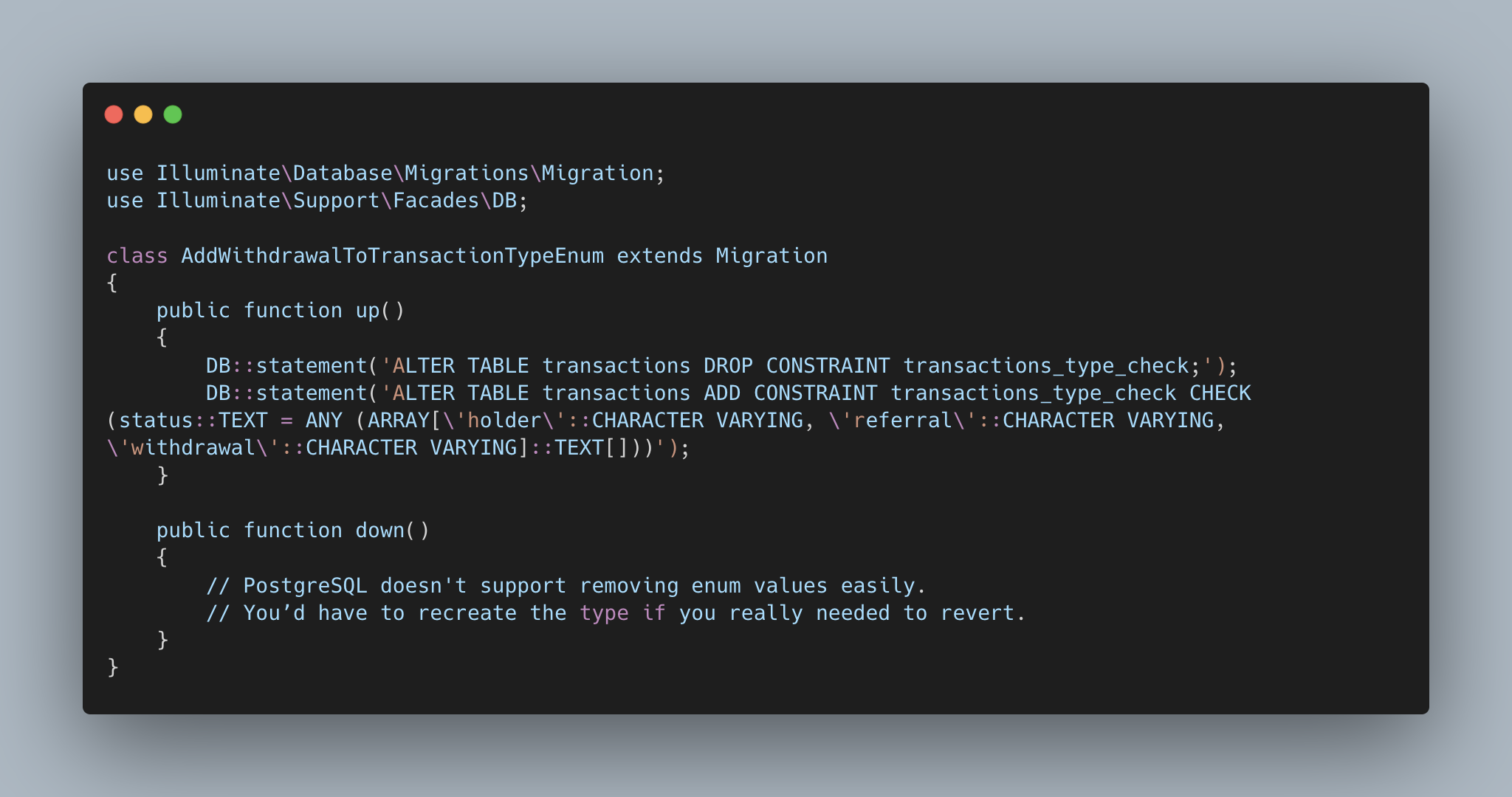
How to Add a New Value to a PostgreSQL ENUM Column in Laravel
If you're using PostgreSQL with Laravel and have a table column defined as an ENUM, you may run into a common issue: PostgreSQL does not let you modify ENUMs like regular fields. Instead, you need to alter the underlying ENUM type directly — which can be confusing at first.
Here’s how to properly add a new value to a PostgreSQL ENUM column in a Laravel project.
Use Case
Let’s say you have a transactions
table with a type
column defined as an ENUM in PostgreSQL. Initially, it supports values like 'credit'
and 'debit'
.
Now you want to add a new option: 'withdrawal'
.
Step 1: Create a Migration to Add a New Value
Create a migration:
php artisan make:migration add_withdrawal_to_transaction_type_enum
Inside the generated file:
use Illuminate\Database\Migrations\Migration;
use Illuminate\Support\Facades\DB;
class AddWithdrawalToTransactionTypeEnum extends Migration
{
public function up()
{
DB::statement('ALTER TABLE transactions DROP CONSTRAINT transactions_type_check;');
DB::statement('ALTER TABLE transactions ADD CONSTRAINT transactions_type_check CHECK (status::TEXT = ANY (ARRAY[\'holder\'::CHARACTER VARYING, \'referral\'::CHARACTER VARYING, \'withdrawal\'::CHARACTER VARYING]::TEXT[]))');
}
public function down()
{
// PostgreSQL doesn't support removing enum values easily.
// You’d have to recreate the type if you really needed to revert.
}
}
Run the migration:
php artisan migrate