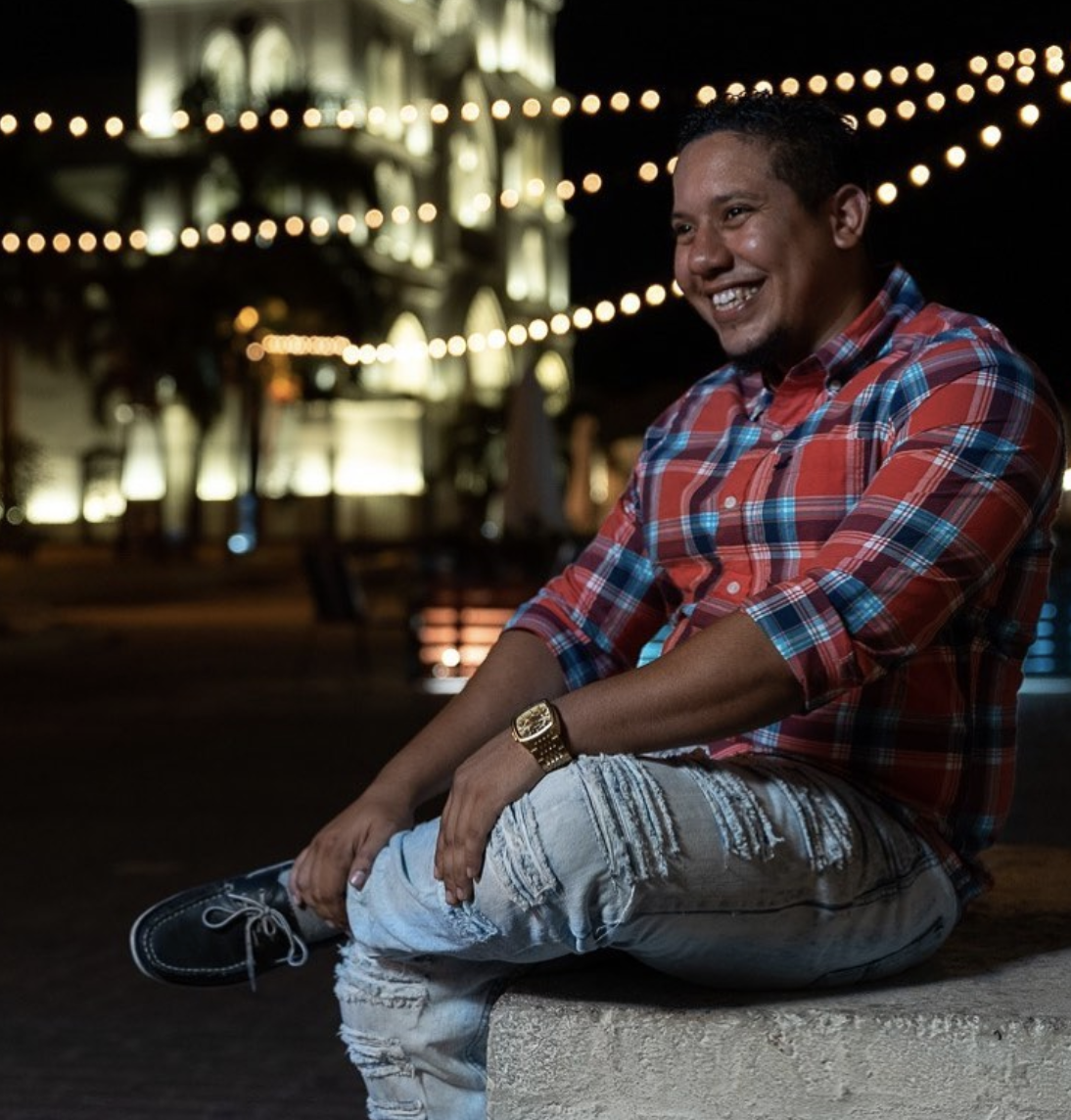
Fermin Perdomo
Full Stack Developer
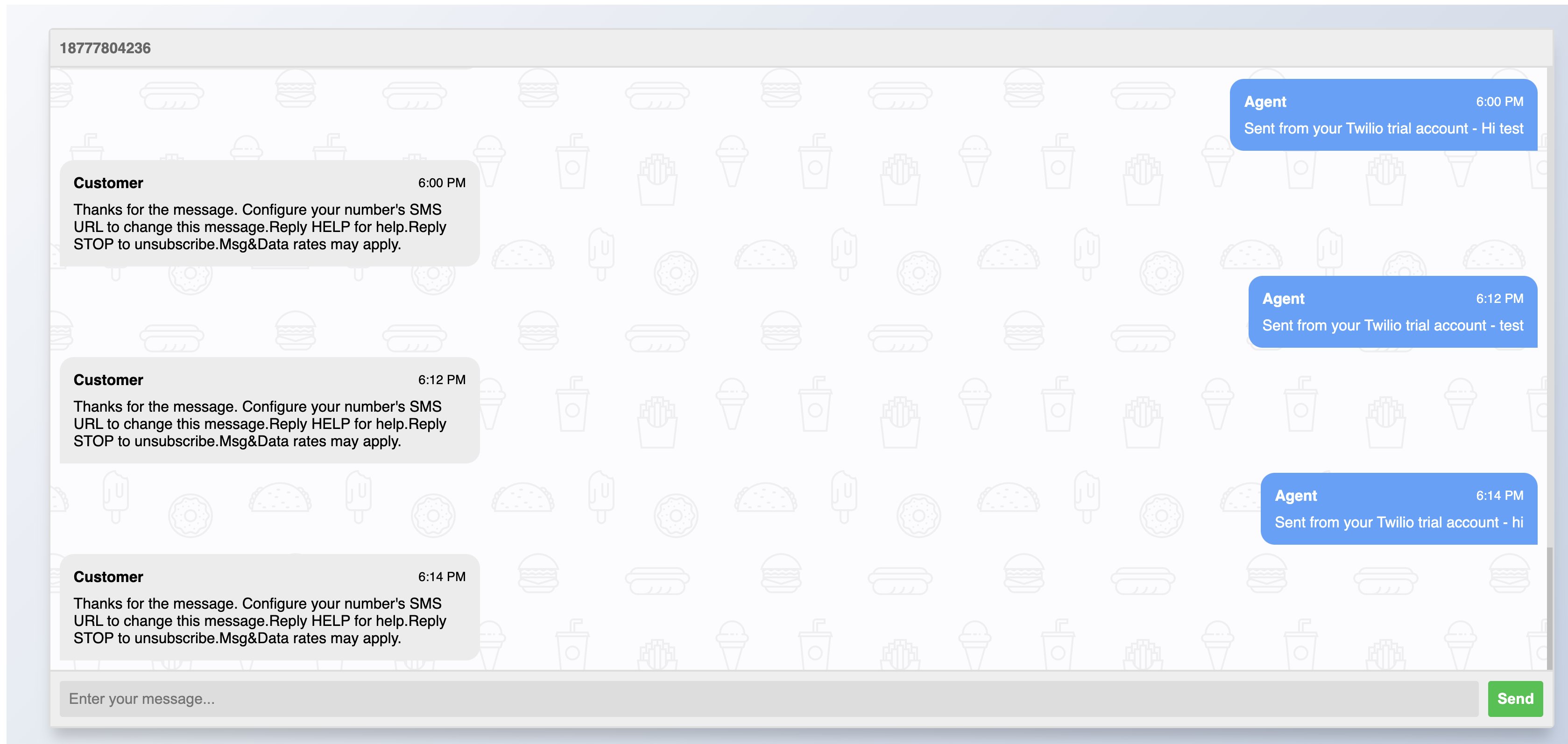
How to Create an SMS Chat System Using PHP and Twilio to Integrate with VICIdial
In today's fast-paced business environment, having an efficient SMS chat system can enhance communication with customers and improve lead conversion. If you're using VICIdial, an open-source contact center solution, integrating an SMS chat system can help you engage with your clients more effectively. In this guide, we will walk you through the process of creating an SMS chat system using PHP and Twilio.
Prerequisites
Before you get started, ensure you have the following:
A Twilio account with a purchased phone number.
A VICIdial installation.
PHP installed on your server.
A web server (Apache/Nginx) with PHP support.
Basic knowledge of PHP and web development.
Step 1: Set Up Your Project
Create a new project directory and set up the following
composer.json
file:{ "name": "masterfermin02/smschat", "description": "Simple php sms chat", "keywords": [ "sms", "chat", "smschat", "vicidial", "Twilio" ], "homepage": "https://github.com/masterfermin02", "license": "MIT", "autoload": { "psr-4": { "FpApp\\": "src/" }, "files": [ "src/helpers.php" ] }, "require": { "twilio/sdk": "^6.9", "vlucas/phpdotenv": "^5.6", "twig/twig": "^3.11", "patricklouys/http": "^1.4", "nikic/fast-route": "^1.3" } }
Run the following command to install dependencies:
composer install
Step 2: Project Structure
Organize your project with the following structure:
project-root/
│-- src/
│ ├-- helpers.php
│ ├-- Env.php
│ ├-- Factories/
│ │ ├-- SmsFactory.php
│ │ ├-- SmsGatewayFactory.php
│ │ ├-- SmsModelFactory.php
│ └-- Middleware/
│ └-- AgentIsOnline.php
│-- resources/
│ └-- views/
│ ├-- chat.twig
│ └-- chat-screen.twig
│-- public/
│ └-- index.php
│-- .env
│-- composer.json
│-- vendor/
Step 3: Handling Incoming SMS
Create a public/index.php
file to process Twilio requests:
require __DIR__ . '/../vendor/autoload.php';
use Dotenv\Dotenv;
use Http\HttpRequest;
use Http\HttpResponse;
use Twig\Environment;
use Twig\Loader\FilesystemLoader;
use FpApp\Factories\SmsFactory;
use Twilio\TwiML\MessagingResponse;
$dotenv = Dotenv::createImmutable(__DIR__ . '/../');
$dotenv->load();
$loader = new FilesystemLoader(__DIR__ . '/../resources/views');
$twig = new Environment($loader);
$request = new HttpRequest($_GET, $_POST, $_COOKIE, $_FILES, $_SERVER, file_get_contents('php://input'));
$response = new HttpResponse();
$response->setHeader('Content-Type', 'application/xml');
$sms = SmsFactory::create();
$sms->handleIncoming($request->getParameter('From'), $request->getParameter('Body'));
$twiml = new MessagingResponse();
$twiml->message("Thank you for your message.");
$response->setContent($twiml);
echo $response->getContent();
Step 4: Sending SMS
Use the following script to send SMS messages:
$sms = SmsFactory::create();
$result = $sms->send('+1234567890', Env::get('TWILIO_PHONE_NUMBER'), 'Hello, this is a test message.');
Step 5: Automating SMS Workflows
You can set up automated workflows for lead follow-ups, appointment reminders, and survey collections using the routing and middleware capabilities of the project.
Conclusion
By structuring your project with PHP and Twilio using modern practices like dependency management and routing, you can create an efficient SMS chat system integrated with VICIdial to improve your customer engagement.