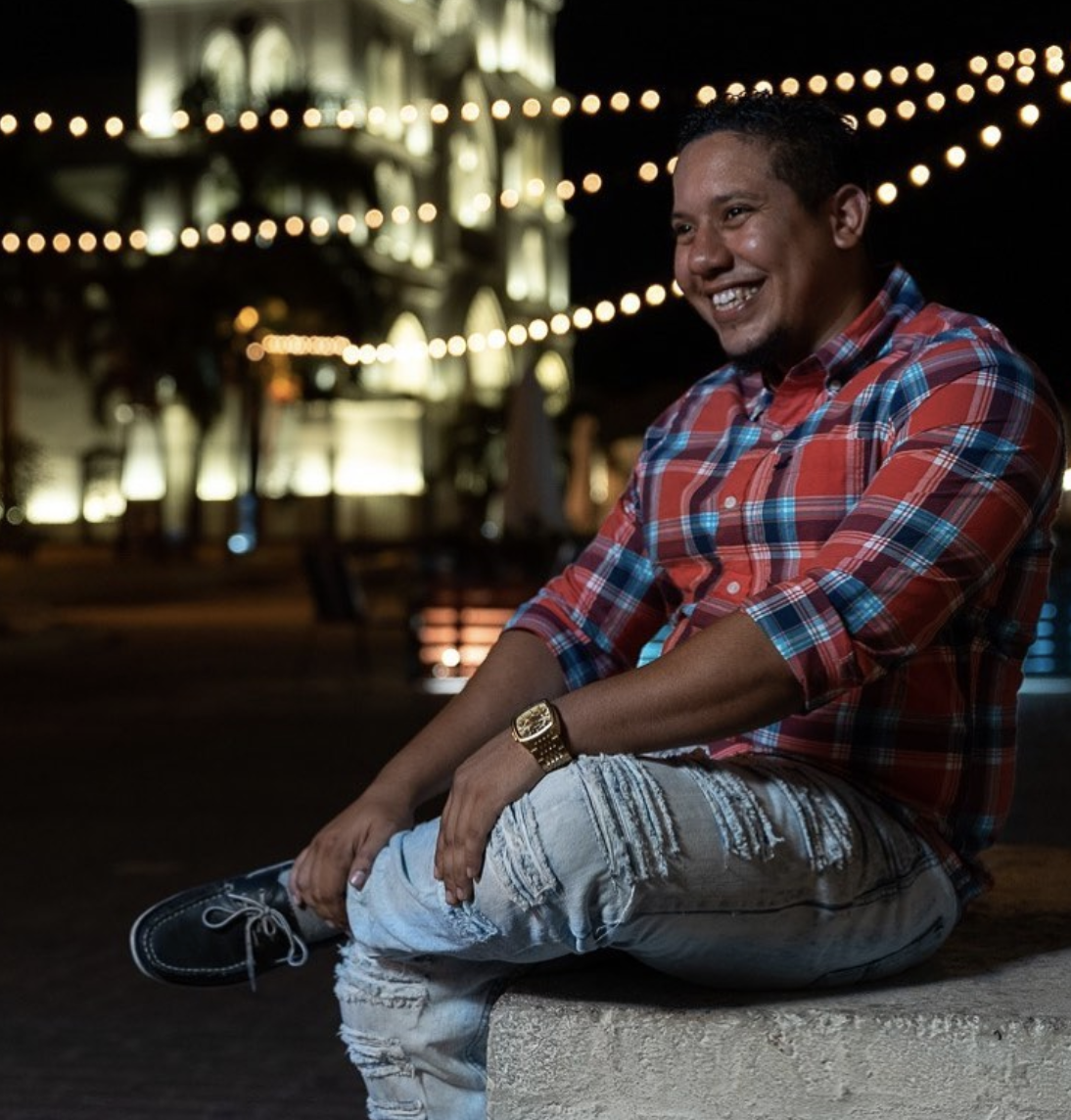
Fermin Perdomo
Full Stack Developer
© 2025 Fermin's Portfolio | All rights reserved.
.png)
How unique an array of object with js by properties
Today, I will explain how to unique an array of object by property
Unique by a Single Property
If you want the objects in the array to be unique based on one property (e.g., id):
const array = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 1, name: 'Alice' }, // Duplicate based on id
];
const uniqueArray = Array.from(
new Map(array.map(item => [item.id, item])).values()
);
console.log(uniqueArray);
// Output: [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }]
Unique by Multiple Properties
If you want uniqueness based on a combination of properties, you can create a unique key by combining the values of those properties:
const array = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 1, name: 'Alice' },
{ id: 1, name: 'Charlie' }, // Same id, different name
];
const uniqueArray = Array.from(
new Map(array.map(item => [`${item.id}-${item.name}`, item])).values()
);
console.log(uniqueArray);
// Output: [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, { id: 1, name: 'Charlie' }]
Using filter
for Simplicity
Alternatively, you can use the filter
method for uniqueness by checking if an item's index matches its first occurrence:
const array = [
{ id: 1, name: 'Alice' },
{ id: 2, name: 'Bob' },
{ id: 1, name: 'Alice' },
];
const uniqueArray = array.filter(
(item, index, self) =>
index === self.findIndex(obj => obj.id === item.id)
);
console.log(uniqueArray);
// Output: [{ id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }]
Explanation
Map and Set: Efficient for large arrays because they use hashing for uniqueness.
filter + findIndex: More readable but can be slower for large arrays due to nested iterations.
Custom Combination Key: Use when uniqueness depends on multiple properties.